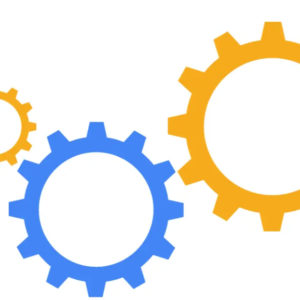
Using Output expressions with the Microsoft Rules engine
The Microsoft Rules engine is a powerful tool to isolate business logic from an application and supports output parameters. Suppose we have a requirement to detect duplicate…
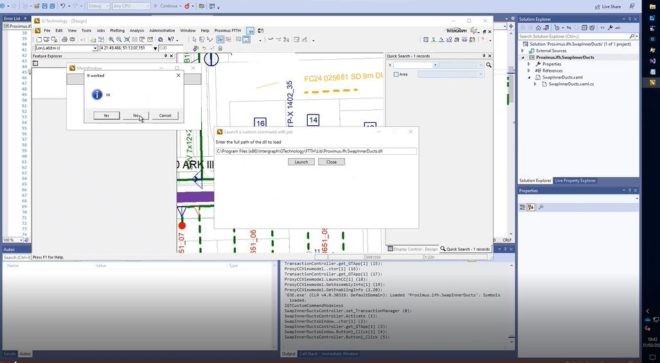
Shortening CustomCommand development time by using a proxy
One of the most time-consuming processes when developing with Hexagon G/Technology is creating custom commands. The usual development approach consists of the following iterative process: During testing,…
Workaround for the Dotnet Maui Tabs ‘OnAppearing’ event
Tabs in dotnet maui have an ‘OnAppearing’ event which you would expect to be called each time a tab is activated. This however is not the case…
A Multilevel Responsive Blazor-menu
When you generate a Blazor-server app or Webassembly the standard template generates a menu docked to the left which is responsive, but has some drawbacks : It…
Invoking Oracle Spatial from a Custom Command
Consider a requirement where the centroid of a set of points needs to be retrieved within a custom command. This can be achieved using the ‘GTDataProvider.DataContext.Execute‘-method:
Rubberbanding in G/Technology
Whenever a user is digitizing a polygon, he expects feedback when moving the cursor before actually adding a point, the system should ‘rubberband’ the position under the…
Specialized ViewComponents
Suppose you want to introduce an inheritance chain in ASP.Net Core to use some businesslogic in a baseclass : ViewComponentSpecialized.Detail renders like this : If you invoke…
Configuring Webservice endpoints in a .Net Core Webapplication
If you need to invoke a WCF-Soap compliant Webservice from an ASP.Net application, the endpoints for it are stored in the Web.Config: If you need to invoke…
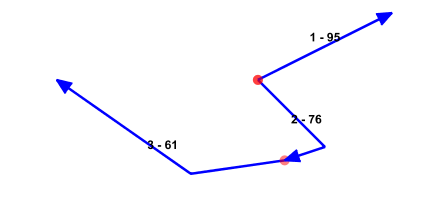
Sorting and merging geometries in Oracle Spatial
Consider a requirement where an unsorted set of geometries needs to be merged into a single geometry. Take for instance the following set of geometries where: a…
SDO_GEOM.VALIDATE_GEOMETRY_WITH_CONTEXT 3D bug workaround
The SDO_GEOM.VALIDATE_GEOMETRY_WITH_CONTEXT function fails for 3D Compound line strings geometries as discussed over here. This is illustrated by the following sample: 2d: produces: While the same geometry…