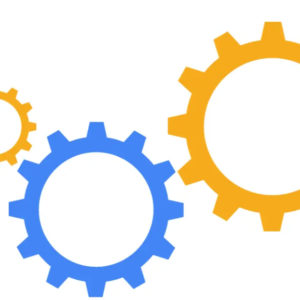
Using Output expressions with the Microsoft Rules engine
The Microsoft Rules engine is a powerful tool to isolate business logic from an application and supports output parameters. Suppose we have a requirement to detect duplicate…
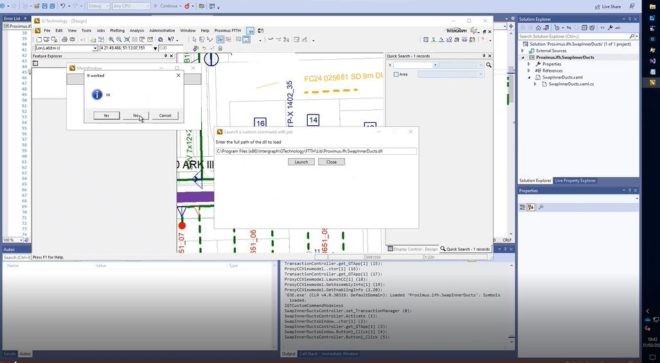
Shortening CustomCommand development time by using a proxy
One of the most time-consuming processes when developing with Hexagon G/Technology is creating custom commands. The usual development approach consists of the following iterative process: During testing,…
Workaround for the Dotnet Maui Tabs ‘OnAppearing’ event
Tabs in dotnet maui have an ‘OnAppearing’ event which you would expect to be called each time a tab is activated. This however is not the case…