Suppose we have a Cable feature with CABLE_TYPE, TOTAL_SIZE and FIBER_SIZE attributes. Whenever the CABLE_TYPE attribute is set, attributes TOTAL_SIZE and FIBER_SIZE need to be set as well. We need to implement the following list :
ID | Cable type | Total size | Fiber Size |
1 | Corning 12F | 12 | 4 |
2 | BICC 16F | 16 | 4 |
3 | BICC 48F | 48 | 4 |
4 | PHILLIP 100F | 100 | 6 |
5 | Corning 48F | 48 | 8 |
6 | Corning 96F | 96 | 10 |
7 | Emtelle 12F | 12 | 12 |
8 | Emtelle 144F | 144 | 12 |
9 | Emtelle 240F | 240 | 12 |
We can turn this list into picklists with the following statement:
create table CABLE_TYPE ( id int primary key, material_id varchar( 20) not null, total_size integer not null, fiber_size integer not null ); insert into cable_type( id, material_id, total_size, fiber_size) values ( 1, 'Corning 12F', 12, 4); insert into cable_type( id, material_id, total_size, fiber_size) values ( 2, 'BICC 16F', 16, 4); insert into cable_type( id, material_id, total_size, fiber_size) values ( 3, 'BICC 48F', 48, 4); insert into cable_type( id, material_id, total_size, fiber_size) values ( 4, 'PHILLIP 100F', 100, 6); insert into cable_type( id, material_id, total_size, fiber_size) values ( 5, 'Corning 48F', 48, 8); insert into cable_type( id, material_id, total_size, fiber_size) values ( 6, 'Corning 96F', 96, 10); insert into cable_type( id, material_id, total_size, fiber_size) values ( 7, 'Emtelle 12F', 12, 12); insert into cable_type( id, material_id, total_size, fiber_size) values ( 8, 'Emtelle 144F', 144, 12); insert into cable_type( id, material_id, total_size, fiber_size) values ( 9, 'Emtelle 240F', 240, 12); insert into G3E_PICKLIST ( G3E_PNO, G3E_USERNAME, G3E_TABLE, G3E_KEYFIELD,G3E_VALUEFIELD, G3E_VALIDATION, G3E_ORDERBYKEY, G3E_PUBLISH) Values( 111, 'Cable Type', 'CABLE_TYPE', 'MATERIAL_ID', 'MATERIAL_ID','G3ERestricted', 'ID',0); insert into G3E_PICKLIST ( G3E_PNO, G3E_USERNAME, G3E_TABLE, G3E_KEYFIELD, G3E_VALUEFIELD, G3E_VALIDATION, G3E_ORDERBYKEY, G3E_PUBLISH) Values( 112,'Total Size', 'CABLE_TYPE', 'TOTAL_SIZE', 'TOTAL_SIZE', 'G3ERestricted','ID',0); insert into G3E_PICKLIST ( G3E_PNO, G3E_USERNAME, G3E_TABLE, G3E_KEYFIELD,G3E_VALUEFIELD, G3E_VALIDATION, G3E_ORDERBYKEY, G3E_PUBLISH) Values( 113, 'Fiber Size', 'CABLE_TYPE', 'FIBER_SIZE', 'FIBER_SIZE', 'G3ERestricted', 'ID', 0);
We can now achieve the desired functionality using ‘Triggersets’. A triggerset is a rule describing a way to set values on a set of given attributes whenever one special attribute (the ‘Triggering’-attribute) changes.
Suppose our attributes have the following characteristics, taken from G3E_ATTRIBUTE :
g3e_ano | g3e_field | g3e_pno |
110206 | CABLE_TYPE | NULL |
110207 | TOTAL_SIZE | 112 |
110208 | FIBER_SIZE | 113 |
And the following, taken from G3E_TABATTRIBUTE :
g3e_tano | g3e_ano | g3e_pno | g3e_triggerpopulate |
110201 | 110206 | 111 | 1 |
110202 | 110207 | NULL | 0 |
110203 | 110208 | NULL | 0 |
There’s only one field with a G3E_TRIGGERPOPULATE value of 1, the ‘Triggering attribute’. This means that whenever this attribute gets a different value, the GTech triggering mechanism kicks off.
Given the above attributes and menu-definition, you can create a triggerset by populating tables G3E_TRIGGERSET & G3E_AUTOPOPULATE :
insert into g3e_triggerset( g3e_tsno, g3e_setno, g3e_triggertano, g3e_triggerfilter) values ( 2, 2, 110201, '(MATERIAL_ID=#110206)');
The syntax in the G3E_TRIGGERFILTER column tells GTech how to retrieve a row from the picklist table to select a single row, to select the values to be used for updating. The values to be updated are specified using G3E_AUTOPOPULATE :
insert into g3e_autopopulate( g3e_apno, g3e_setno, g3e_tano ) values ( 3, 2, 110202 ); insert into g3e_autopopulate( g3e_apno, g3e_setno, g3e_tano ) values ( 4, 2, 110203 );
The first statement tells GTech to update the attribute on the dialog with g3e_tano 110202 whenever the value for CABLE_TYPE changes. The value will be the value for picklist 112 t.i. CABLE_TYPE.TOTAL_SIZE, as defined in G3E_ATTRIBUTE. Note that for the autopopulate mechanism to work, you need to have your picklist defined on G3E_ATTRIBUTE.G3E_PNO, not G3E_TABATTRIBUTE.G3E_PNO.
The second statement tells GTech to update g3e_tano 110203 with the value found in picklist 113, which is column CABLE_TYPE.FIBER_SIZE.
When this all is set in place, you’ll get the following initial picture :
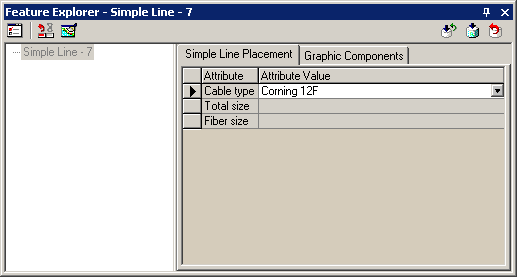
If you select ‘Cable Type’ the following drop down shows up :
If the ‘Cable Type’ field has lost focus the triggerset fires and you’ll see the following screen
Once you select field ‘Cable type’ and then leave it, fields ‘Total size’ and ‘Fiber size’ are set.
As you can see, fields Total Size’ & ‘Fiber size’ are made read-only, if the user would be able to change them it would be possible to select invalid cable types.
We now have triggersets in place, but one improvement would be some kind of visual indication for a user what values will be copied into our ‘Total Size’ & ‘Fiber Size’ attributes. This can be done using the following statement:
update g3e_picklist set G3E_ADDITIONALFIELDS = 'TOTAL_SIZE,FIBER_SIZE' where g3e_pno = 111;
If you now select the ‘Cable type’-field, you get a picklist with all the values that will be set using the selected value:
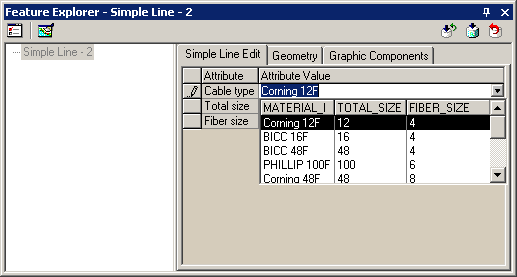
Summary
- Triggersets can be used to set multiple codelisted attributes using a single selection
- All these attributes used in triggersets need to be defined in the same picklist table
Notes
- The same functionallity could also be arranged with functional interfaces, but that would require developing custom code, Triggersets are part of the Product
- Triggersets & autopopulate values need to use columns from the same picklist. If one attribute for instance uses a column from a different picklist, you will get the following error
Triggerset error - Triggersets cannot be used accross different components. If you need to set an attribute in a different component, you need to do it using functional interfaces
Hope this helps, Stephan