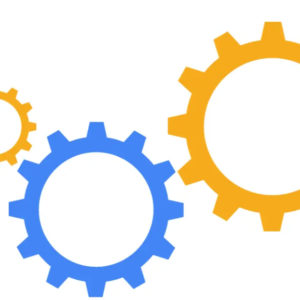
Using Output expressions with the Microsoft Rules engine
The Microsoft Rules engine is a powerful tool to isolate business logic from an application and supports output parameters. Suppose we have a requirement to detect duplicate…
Specialized ViewComponents
Suppose you want to introduce an inheritance chain in ASP.Net Core to use some businesslogic in a baseclass : ViewComponentSpecialized.Detail renders like this : If you invoke…
Configuring Webservice endpoints in a .Net Core Webapplication
If you need to invoke a WCF-Soap compliant Webservice from an ASP.Net application, the endpoints for it are stored in the Web.Config: If you need to invoke…