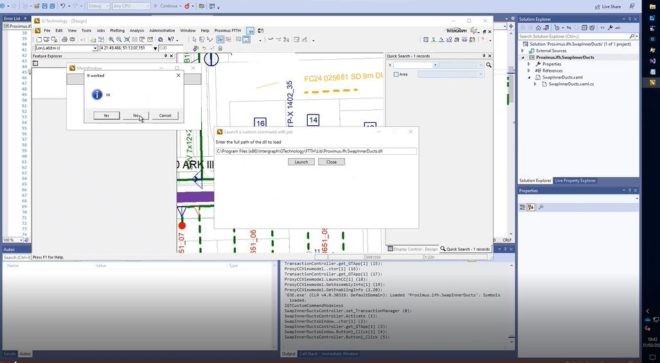
Shortening CustomCommand development time by using a proxy
One of the most time-consuming processes when developing with Hexagon G/Technology is creating custom commands. The usual development approach consists of the following iterative process: During testing,…
A Multilevel Responsive Blazor-menu
When you generate a Blazor-server app or Webassembly the standard template generates a menu docked to the left which is responsive, but has some drawbacks : It…